by Riddhima Singh
4098
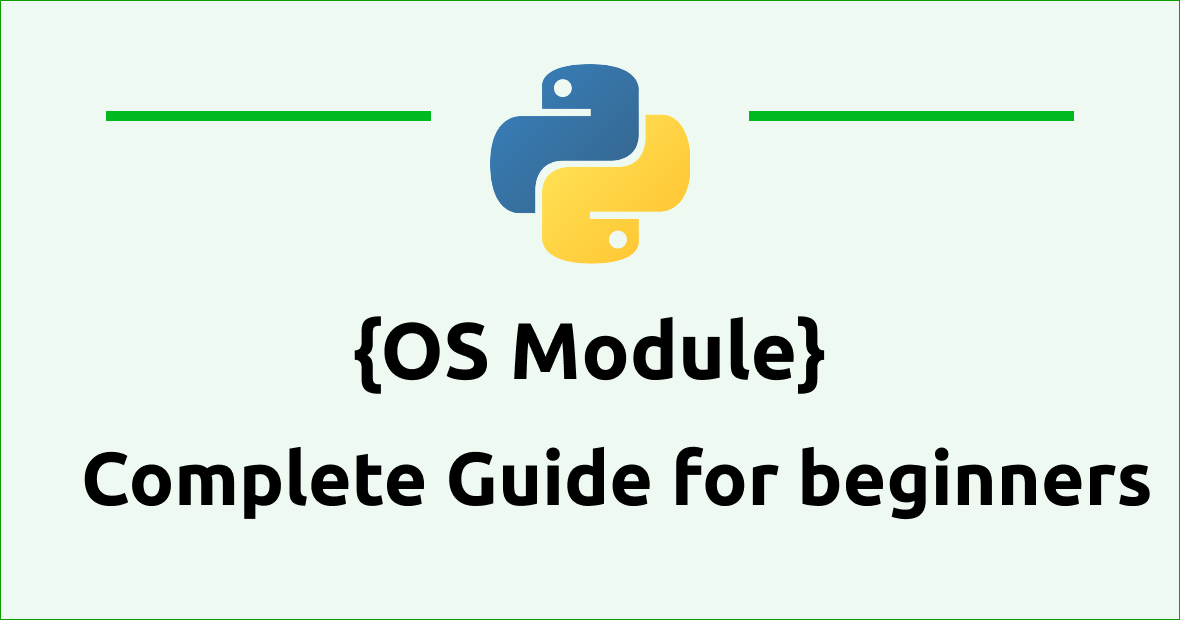
Introduction to OS Module
As we all know Python is very popular for its large standard libraries. And it has a module called OS
A module that provides runtime interaction with the operating system for creating, removing, fetching, changing, and identifying directories files and paths.
Table of Contents
Getting started with OS Module in Python
Installation
OS is a part of Python's default standard libraries so don't require to install just import os
before using its functions and you are done. The OS module is one of Python's standard utilities🤖.
import os
Common function of the OS Module
os.mkdir()
To create new directories by using os.mkdir().
it takes a single argument which is the name of new directory.
import os
os.mkdir("newdir")
The function creates a new directory named newdir in the current working directory.
os.getcwd()
os.getcwd()
returns the current working directory (CWD) of your current location where your Python script is running.
import os
print(os.getcwd())
os.chdir()
We can use os.chdir()
it to change your current working directory. This function takes a single argument which will be your CWD.
import os
os.chdir("/new/directory")
os.rmdir()
It is possible to remove the specified directory with an absolute path using the os.rmdir()
function. This function also takes a single argument.
import os
os.rmdir("/path/to/directory")
os.listdir()
os.listdir()
is used to get a list of all files and directories in your current working directory.
list_of_files = os.listdir()
print(list_of_files)
os.error()
When invalid or inaccessible file names or paths are encountered, os.error()
raises an OSError.
import os
try:
filename = 'os_python.txt'
f = open(filename, 'r')
text = f.read()
f.close()
except IOError:
print(' Reading : ' + filename)
os.popen()
A file object attached to a pipe is returned by os.popen()
, which opens a file or runs a command.
import os
new_file = "python.txt"
# popen() is similar to open()
file = open(new_file, 'w')
file.write("Welcome!To codeasify ")
file.close()
file = open(new_file, 'r')
text = file.read()
print(text)
# popen() provides gateway and accesses the file directly
file = os.popen(new_file, 'w')
file.write("This is awesome")
os.close()
Files associated with descriptor fr are closed with this function.
import os
new_file = "Python1.txt"
file = open(new_file, 'r')
text = file.read()
print(text)
os.close(file)
os.rename()
os.rename()
is used to rename files. If the user has the privilege to change a file. It takes two arguments.
import os
os.rename("old_name", "new_name")
os.access()
The invoking user's uid and gid are used to test if the path is accessible.
import os
import sys
path_1 = os.access("Python.txt", os.F_OK)
print("Exist path:", path_1)
# Checking access with os.R_OK
path_2 = os.access("Python.txt", os.R_OK)
print("It access to read the file:", path_2)
# Checking access with os.W_OK
path_3 = os.access("Python.txt", os.W_OK)
print("It access to write the file:", path_3)
# Checking access with os.X_OK
path_4 = os.access("Python.txt", os.X_OK)
print("Check if path can be executed:", path_4)
Working with file paths with OS module in Python
os.path.join()
os.path.join()
is helpful to concatenate multiple strings to create a valid path. Follow the given example.
import os
path = os.path.join('directory', 'subdirectory', 'filename')
print(path)
os.path.split()
os.path.split() is used to separate a file name and the directory. this function takes the complete file path and split it into two parts one is head and the second is the tail. Follow the given example.
head, tail = os.path.split('/directory/subdirectory/file.txt')
print(head) # Output: /directory/subdirectory
print(tail) # Output: file.txt
os.path.exists()
os.path.exists()
checks whether a mentioned file name exists or not. if it exists then it returns True
if not then it returns False.
if os.path.exists('file.txt'):
print("The file exists!")
else:
print("The file does not exist.")
os.path.isdir()
os.path.isdir()
checks whether a mentioned path is a directory or not. if it exists then it returns True
if not then it returns False.
if os.path.isdir('file.txt'):
print("is directory")
else:
print("not a directory")
os.path.isfile()
os.path.isfile()
checks whether a mentioned path is a file or not. if it exists then it returns True
if not then it returns False.
if os.path.isfile('file.txt'):
print("is file!")
else:
print("not a file")
Environment variables set by OS packages
Programs running on a system can access and modify environment variables♻️ using the environment attribute in the OS package. Environment variables can be manipulated in several ways with the OS package:
👉 Getting Environment Variables: For instance, to retrieve the value of the PATH environment variable, use the method os.environ.get()
:
import os
path = os.environ.get('PATH')
print(path)
👉 Configuring Environment Variables: The os.environ()
dictionary allows you to set an environment variable's value. For example, the following code sets the value of var to "hello":
import os
os.environ['var'] = 'hello'
You can only modify the environment variables for the current Python process and any child processes it creates by using os.environ
.
👉 Deleting Environment Variables: You can delete an environment variable using the os.environ.pop()
method For example, to delete the var environment variable, you can use the following code:
import os
os.environ.pop(‘var’, None)
It is returned by pop()
method if the environment variable has been removed. If the environment variable does not exist, the value of None is returned.
👉 List All Environment Variables: Using the os.environ.items()
method, you can see the values of all environment variables. For example, you can do the following:
import os
for key, value in os.environ.items():
print(key, value)
Conclusion
A platform-independent way 🛣️ of interacting with the operating system is provided by the os module in Python. It provides functions for handling files, directories, environment variables, and processes. Python code written with the os module works seamlessly on different platforms.
Hope you guys enjoy 😊 it.